PHP Variables:
As with algebra, PHP variables can be used to hold values (x=5) or expressions (z=x+y).
Variable can have short names (like x and y) or more descriptive names (age, carname, totalvolume).
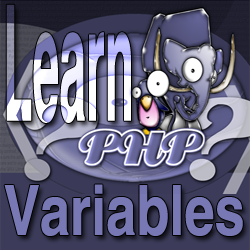
Naming Conventions
Variables in PHP must abide by certain naming conventions. Specifically, they
- start with the dollar sign
- must contain only letters, numbers, and the underscore
- cannot start with a number
- are case sensitive
This last rule can trip up many programmers as $var and $Var are two separate variables. To minimize confusion and errors, it’s best to stick to one naming scheme. The two most common styles are
- Use all lowercase, separating words with underscores: $my_var, $first_name, etc.
- Use primarily lowercase, separating words with capital letters: $myVar, $firstName, etc.
Rules for PHP variables:
- A variable starts with the $ sign, followed by the name of the variable
- A variable name must begin with a letter or the underscore character
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
- A variable name should not contain spaces
- Variable names are case sensitive ($y and $Y are two different variables)
Example of Variable
<!DOCTYPE html>
<html>
<body>
<?php
$x=5;
$y=6;
$z=$x+$y;
echo $z;
?>
</body>
</html>
PHP String Variables
A string variable is used to store and manipulate text.
String Variables in PHP
String variables are used for values that contain characters.
After we have created a string variable we can manipulate it. A string can be used directly in a function or it can be stored in a variable.
In the example below, we create a string variable called txt, then we assign the text “Hello world!” to it. Then we write the value of the txt variable to the output:
Example:
<!DOCTYPE html>
<html>
<body>
<?php
$txt=”Hello world!”;
echo $txt;
?>
</body>
</html>
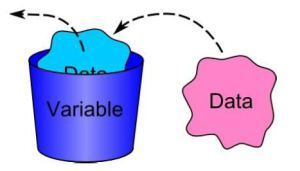
PHP Variable Scopes
The scope of a variable is the part of the script where the variable can be referenced/used.
PHP has four different variable scopes:
- local
- global
- static
Local Scope
A variable declared within a PHP function is local and can only be accessed within that function:
Example:
<!DOCTYPE html>
<html>
<body>
<?php
$x=5; // global scope
function myTest()
{
echo $x; // local scope
}
myTest();
?>
</body>
</html>
Global Scope:
First, an example use of global:
Example #1 Using global
<?php
$a = 1;
$b = 2;
function Sum(){
global $a, $b;
$b = $a + $b;
}
Sum();
echo $b;
?>
The above script will output 3. By declaring $a and $b global within the function, all references to either variable will refer to the global version. There is no limit to the number of global variables that can be manipulated by a function.
Note:
Using global keyword outside a function is not an error. It can be used if the file is included from inside a function.
Static Scope:
Another important feature of variable scoping is the static variable. A static variable exists only in a local function scope, but it does not lose its value when program execution leaves this scope. Consider the following example:
Example #4 Example demonstrating need for static variables
<?php
function test()
{
$a = 0;
echo $a;
$a++;
}
?>
This function is quite useless since every time it is called it sets $a to 0 and prints 0. The $a++ which increments the variable serves no purpose since as soon as the function exits the $a variable disappears. To make a useful counting function which will not lose track of the current count, the $a variable is declared static:
Example #5 Example use of static variables
<?php
function test()
{
static $a = 0;
echo $a;
$a++;
}
?>
Now, $a is initialized only in first call of function and every time the test() function is called it will print the value of $a and increment it.
Static variables also provide one way to deal with recursive functions. A recursive function is one which calls itself. Care must be taken when writing a recursive function because it is possible to make it recurse indefinitely. You must make sure you have an adequate way of terminating the recursion. The following simple function recursively counts to 10, using the static variable $count to know when to stop: